Financial model
Corda includes a standard library of data types used in financial applications and contract state objects. These provide a common language for states and contracts.
Glossary
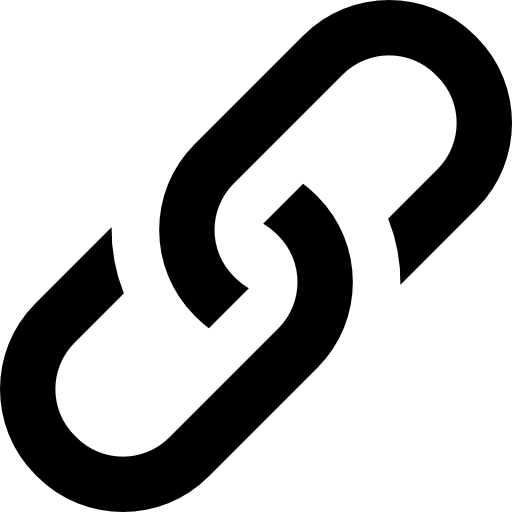
Fungible
If you could exchange an asset for an identical asset, and the asset can be split and merged, then it is fungible. For example, US dollars are fungible because you could exchange a $5 bill for another $5 bill, which you could break down into five $1 bills. However, there is only one Mona Lisa, which cannot be subdivided - so the Mona Lisa is not a fungible asset.
Token
A type used to define the underlying financial product in a transaction.
Amount
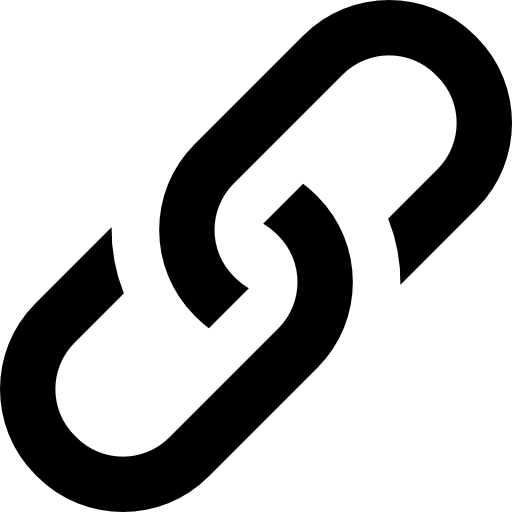
The Amount class represents an amount of
a fungible asset. It is a generic class which wraps around the token. For example, the Amount
could be:
- The standard JDK type
Currency
. - An
Issued
instance. - A more complex asset type, such as an obligation contract issuance definition. The issuance definition contains a token definition, which defines the currency the obligation must be settled in. Custom token types should implement
TokenizableAssetInfo
to allow theAmount
conversion helpersfromDecimal
andtoDecimal
to calculate the correctdisplayTokenSize
.
Here are some examples:
// A quantity of some specific currency like pounds, euros, dollars etc.
Amount<Currency>
// A quantity of currency that is issued by a specific issuer, for instance central bank vs other bank dollars
Amount<Issued<Currency>>
// A quantity of a product governed by specific obligation terms
Amount<Obligation.Terms<P>>
Amount
represents quantities as integers. You cannot use Amount
to represent negative quantities
or fractional quantities—use a different type, typically BigDecimal
.
For currencies, the quantity represents the smallest integer amount for that currency, such as cents.
Other assets are more flexible—the quantity could be 1,000 tons of coal or kilowatt hours. You define how the amounts display using the precise conversion ratio in the displayTokenSize
property, which is the BigDecimal
numeric representation of
a single token. Amount
also defines methods to perform overflow/underflow-checked addition and subtraction.
These are operator overloads in Kotlin—you can use them as regular methods from Java). Perform more complex calculations in BigDecimal
, then convert them back to Amount
to make sure the rounding and token conservation works as expected.
Issued
refers to any countable product—for example, cash, a cash-like item, or assets—and an associated PartyAndReference
that describes the issuer of that product.
An issued product typically follows a lifecycle which includes issuance, movement, and an exit from the ledger (for example,
see the Cash
contract and its associated state and commands).
To represent movements of Amount
tokens use the AmountTransfer
type, which records the quantity and perspective
of a transfer. Positive values will indicate a movement of tokens from a source
, for example, a Party
or CompositeKey
to a destination
. Negative values can be used to indicate a retrograde motion of tokens from destination
to source
. AmountTransfer
supports addition (as a Kotlin operator, or Java method) to provide netting
and aggregation of flows. The apply
method can be used to process a list of attributed Amount
objects in a
List<SourceAndAmount>
to carry out the actual transfer.
Financial states
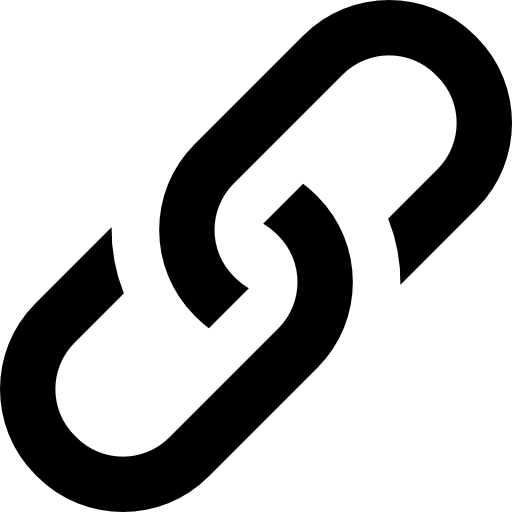
In addition to the common state types, several interfaces extend ContractState
to model financial states such as:
LinearState
: A state that has a unique identifier beyond itsStateRef
, which it carries through state transitions.LinearState
s cannot be duplicated, merged, or split in a transaction: only continued or deleted. UseLinearState
s to model non-fungible items like a specific deal, or an asset that can’t be split, like an airplane.DealState
: ALinearState
representing an agreement between two or more parties. Intended to simplify implementing generic protocols that manipulate many agreement types.FungibleAsset
: Used for contract states that represent countable, fungible assets issued by a specific party. States contain assets which are equivalent (such as cash of the same currency), so records of their existence can be merged or split as needed where the issuer is the same. For example, US dollars issued by the Federal Reserve are fungible and countable (in cents), barrels of oil are fungible and countable (oil from two small containers can be poured into one large container), shares of the same class in a specific company are fungible and countable, and so on. This diagram illustrates the complete contract state hierarchy:
Was this page helpful?
Thanks for your feedback!
Chat with us
Chat with us on our #docs channel on slack. You can also join a lot of other slack channels there and have access to 1-on-1 communication with members of the R3 team and the online community.
Propose documentation improvements directly
Help us to improve the docs by contributing directly. It's simple - just fork this repository and raise a PR of your own - R3's Technical Writers will review it and apply the relevant suggestions.
We're sorry this page wasn't helpful. Let us know how we can make it better!
Chat with us
Chat with us on our #docs channel on slack. You can also join a lot of other slack channels there and have access to 1-on-1 communication with members of the R3 team and the online community.
Create an issue
Create a new GitHub issue in this repository - submit technical feedback, draw attention to a potential documentation bug, or share ideas for improvement and general feedback.
Propose documentation improvements directly
Help us to improve the docs by contributing directly. It's simple - just fork this repository and raise a PR of your own - R3's Technical Writers will review it and apply the relevant suggestions.