CorDapp constraints migration
Corda 4 introduces and recommends building signed CorDapps that issue states with signature constraints. When building transactions in Corda 4, existing on-ledger states that were issued before Corda 4 are only automatically transitioned to the new Signature Constraint if they were originally using the CZ Whitelisted Constraint. Here, we explain how to modify existing CorDapp flows to explicitly consume and evolve pre Corda 4 states.
Upgrading from a Corda 3.x CorDapp to Corda 4
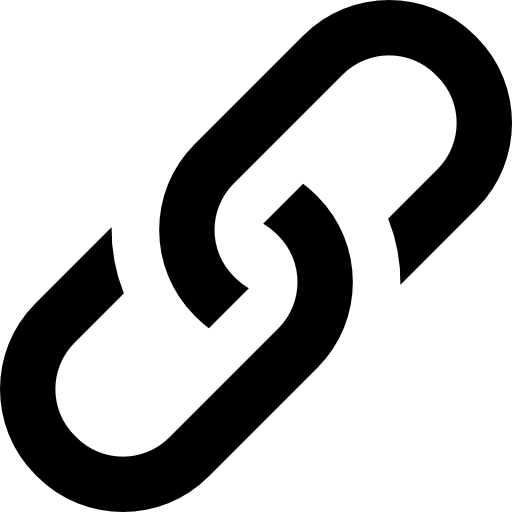
Faced with the exercise of upgrading an existing Corda 3.x CorDapp to Corda 4, you need to consider the following.
What existing unconsumed states have been issued on ledger by a previous version of this CorDapp and using other constraint types?
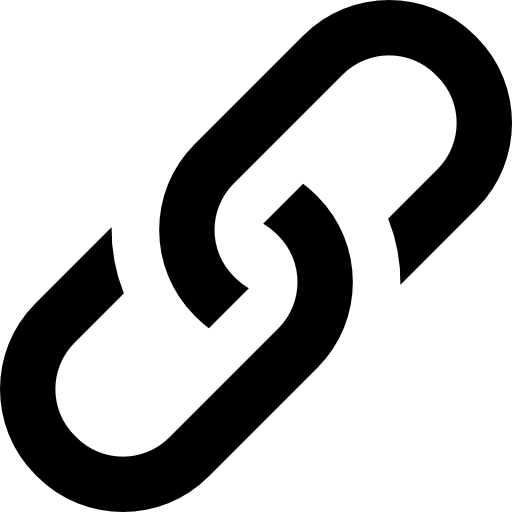
If you have existing hash constrained states, see Migrating hash constraints.
If you have existing CZ whitelisted constrained states, see Migrating CZ whitelisted constraints.
If you have existing always accept constrained states, these are not consumable nor evolvable as they offer no security and should only be used in test environments.
What type of contract states does my CorDapp use?
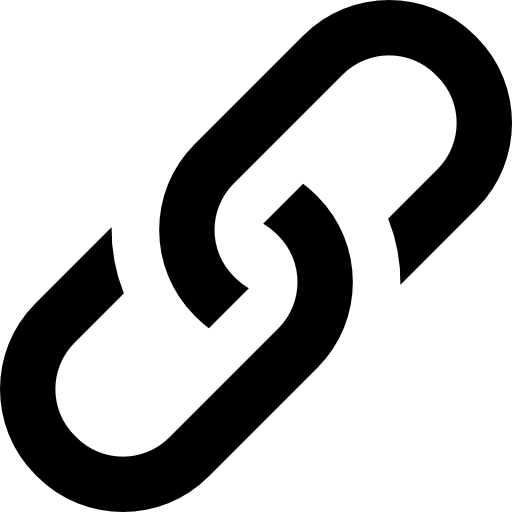
Linear states typically evolve over an extended period of time (defined by the lifecycle of the associated business use case), and thus are prime candidates for constraints migration.
Fungible states are created by an issuer and transferred around a Corda network until explicitly exited (by the same issuer). They do not evolve as linear states, but are transferred between participants on a network. Their consumption may produce additional new output states to represent adjustments to the original state (for example, change when spending cash).
For the purposes of constraints migration, it is desirable that any new output states are produced using the new Corda 4 signature constraint types. Where you have long transaction chains of fungible states, it may be advisable to send them back to the issuer for re-issuance (this is called “chain snipping” and has performance advantages as well as simplifying constraints type migration).
Should I use the implicit or explicit upgrade path?
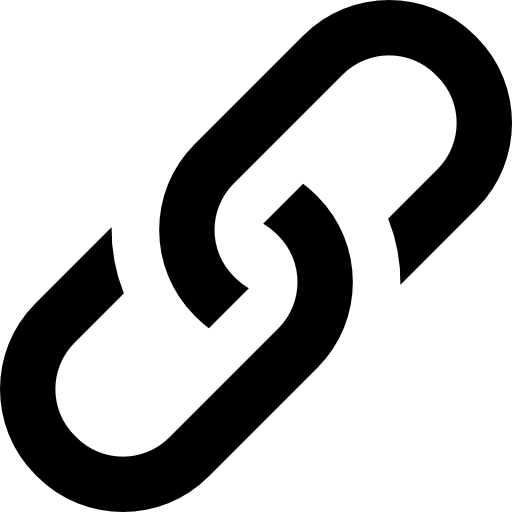
The general recommendation for Corda 4 is to use implicit upgrades for the reasons described here. Implicit upgrades allow pre-authorising multiple implementations of the contract ahead of time. They do not require additional coding and do not incur a complex choreographed operational upgrade process.
Please also remember that states are always consumable if the version of the CorDapp that issued (created) them is installed. In the simplest of scenarios it may be easier to re-issue existing hash or CZ whitelist constrained states (eg. exit them from the ledger using the original unsigned CorDapp and re-issuing them using the new signed CorDapp).
Migrating hash constraints
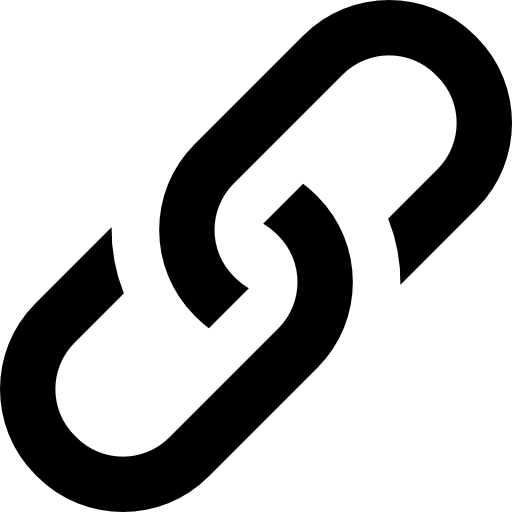
Corda 4.8
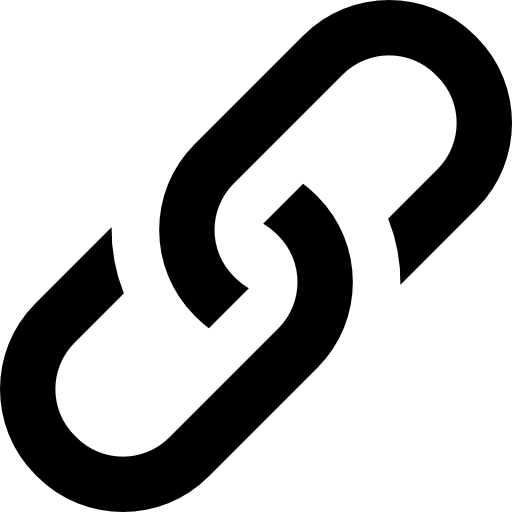
Corda 4.8 requires some additional steps to consume and evolve pre-existing on-ledger hash constrained states:
- All Corda Nodes in the same CZ or business network that may encounter a transaction chain with a hash constrained state must be started using relaxed hash constraint checking mode as described in Hash constrained states in private networks.
- CorDapp flows that build transactions using pre-existing hash-constrained states must explicitly set output states to use signature constraints and specify the related public key(s) used in signing the associated CorDapp Contract JAR:
// This will read the signers for the deployed CorDapp.
val attachment = this.serviceHub.cordappProvider.getContractAttachmentID(contractClass)
val signers = this.serviceHub.attachments.openAttachment(attachment!!)!!.signerKeys
// Create the key that will have to pass for all future versions.
val ownersKey = signers.first()
val txBuilder = TransactionBuilder(notary)
// Set the Signature constraint on the new state to migrate away from the hash constraint.
.addOutputState(outputState, constraint = SignatureAttachmentConstraint(ownersKey))
// This will read the signers for the deployed CorDapp.
SecureHash attachment = this.getServiceHub().getCordappProvider().getContractAttachmentID(contractClass);
List<PublicKey> signers = this.getServiceHub().getAttachments().openAttachment(attachment).getSignerKeys();
// Create the key that will have to pass for all future versions.
PublicKey ownersKey = signers.get(0);
TransactionBuilder txBuilder = new TransactionBuilder(notary)
// Set the Signature constraint on the new state to migrate away from the hash constraint.
.addOutputState(outputState, myContract, new SignatureAttachmentConstraint(ownersKey))
- As a node operator you need to add the new signed version of the contracts CorDapp to the
/cordapps
folder together with the latest version of the flows jar. Please also ensure that the original unsigned contracts CorDapp is removed from the/cordapps
folder (this will already be present in the node’s attachments store) to ensure the lookup code in step 2 retrieves the correct signed contract CorDapp JAR.
Migrating CZ whitelisted constraints
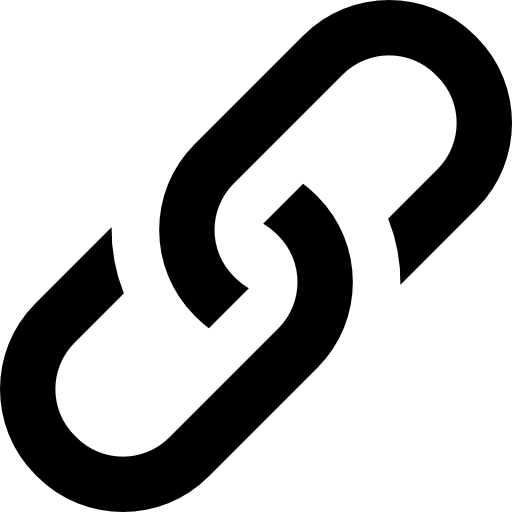
Corda 4.5
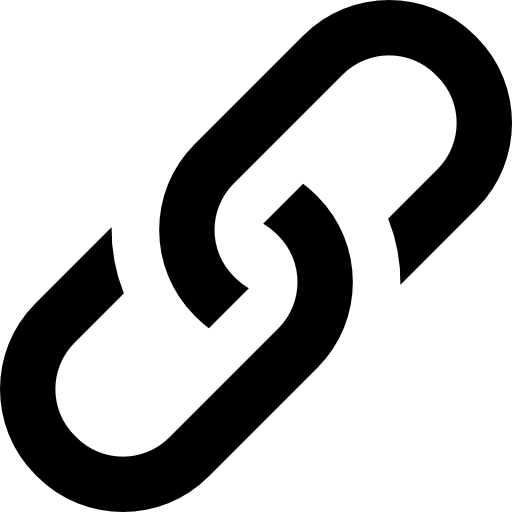
Corda 4.5 requires some additional steps to consume and evolve pre-existing on-ledger CZ whitelisted constrained states:
As the original developer of the CorDapp, the first step is to sign the latest version of the JAR that was released (see Building and installing a CorDapp). The key used for signing will be used to sign all subsequent releases, so it should be stored appropriately. The JAR can be signed by multiple keys owned by different parties and it will be expressed as a
CompositeKey
in theSignatureAttachmentConstraint
(See API: Core types).The new Corda 4 signed CorDapp JAR must be registered with the CZ network operator (as whitelisted in the network parameters which are distributed to all nodes in that CZ). The CZ network operator should check that the JAR is signed and not allow any more versions of it to be whitelisted in the future. From now on the development organisation that signed the JAR is responsible for signing new versions.The process of CZ network CorDapp whitelisting depends on how the Corda network is configured:
If using a hosted CZ network (such as The Corda Network or UAT Environment) running an Identity Operator (formerly known as Doorman) and Network Map Service, you should manually send the hashes of the two JARs to the CZ network operator and request these be added using their network parameter update process.
If using a local network created using the Network Bootstrapper tool, please follow the instructions in Updating the contract whitelist for bootstrapped networks to can add both CorDapp Contract JAR hashes.
Any flow that builds transactions using this CorDapp will automatically transition states to use the
SignatureAttachmentConstraint
if no other constraint is specified and the CorDapp continues to be whitelisted. Therefore, there are two ways to alter the existing code.- Do not specify a constraint
- Explicitly add a Signature Constraint
The code below details how to explicitly add a Signature Constraint:
// This will read the signers for the deployed CorDapp.
val attachment = this.serviceHub.cordappProvider.getContractAttachmentID(contractClass)
val signers = this.serviceHub.attachments.openAttachment(attachment!!)!!.signerKeys
// Create the key that will have to pass for all future versions.
val ownersKey = signers.first()
val txBuilder = TransactionBuilder(notary)
// Set the Signature constraint on the new state to migrate away from the WhitelistConstraint.
.addOutputState(outputState, constraint = SignatureAttachmentConstraint(ownersKey))
// This will read the signers for the deployed CorDapp.
SecureHash attachment = this.getServiceHub().getCordappProvider().getContractAttachmentID(contractClass);
List<PublicKey> signers = this.getServiceHub().getAttachments().openAttachment(attachment).getSignerKeys();
// Create the key that will have to pass for all future versions.
PublicKey ownersKey = signers.get(0);
TransactionBuilder txBuilder = new TransactionBuilder(notary)
// Set the Signature constraint on the new state to migrate away from the WhitelistConstraint.
.addOutputState(outputState, myContract, new SignatureAttachmentConstraint(ownersKey))
- As a node operator you need to add the new signed version of the contracts CorDapp to the
/cordapps
folder together with the latest version of the flows jar. Please also ensure that the original unsigned contracts CorDapp is removed from the/cordapps
folder (this will already be present in the nodes attachments store) to ensure the lookup code in step 3 retrieves the correct signed contract CorDapp JAR.
Was this page helpful?
Thanks for your feedback!
Chat with us
Chat with us on our #docs channel on slack. You can also join a lot of other slack channels there and have access to 1-on-1 communication with members of the R3 team and the online community.
Propose documentation improvements directly
Help us to improve the docs by contributing directly. It's simple - just fork this repository and raise a PR of your own - R3's Technical Writers will review it and apply the relevant suggestions.
We're sorry this page wasn't helpful. Let us know how we can make it better!
Chat with us
Chat with us on our #docs channel on slack. You can also join a lot of other slack channels there and have access to 1-on-1 communication with members of the R3 team and the online community.
Create an issue
Create a new GitHub issue in this repository - submit technical feedback, draw attention to a potential documentation bug, or share ideas for improvement and general feedback.
Propose documentation improvements directly
Help us to improve the docs by contributing directly. It's simple - just fork this repository and raise a PR of your own - R3's Technical Writers will review it and apply the relevant suggestions.